Preventing Port Conflicts in Docker Compose with Dynamic Ports
Learn how to prevent port conflicts in Docker Compose by using dynamic ports instead of hardcoded ones. Improve your local development and CI workflows with this practical DevOps tip.
In DevOps workflows, docker-compose
is an essential tool for spinning up local development environments and orchestrating multi-container applications. But one common issue that creeps up, especially in teams or CI pipelines, is port conflicts. If you’ve ever tried to run two services that both expose port 3000
, you know the pain.
The Problem: Hardcoded Ports
A common docker-compose.yaml
might look like this:
services:
adminer:
image: adminer
restart: always
ports:
- 8080:8080
This configuration maps the container’s port 3000
to the host’s port 3000
. It works until another container or local process already uses that host port. You get a dreaded error like:
Error response from daemon: driver failed programming external connectivity on endpoint port-conflict-adminer-1 (6f1c3c83d8eac300359216fda97536fa4c459577e2f6d802f51a7914fa5fec9f): Bind for 0.0.0.0:8080 failed: port is already allocated
The Better Way: Use Dynamic Host Ports
Instead of hardcoding the host port, let Docker assign an available one dynamically:
services:
adminer:
image: adminer
restart: always
ports:
- 8080
In this case, Docker will map the container's port 3000
to a random available port on the host. This avoids conflicts entirely.
You can inspect the dynamically assigned port with:
docker-compose port adminer 8080
Which might return something like:
0.0.0.0:32768
Now you can open the website with this URL.
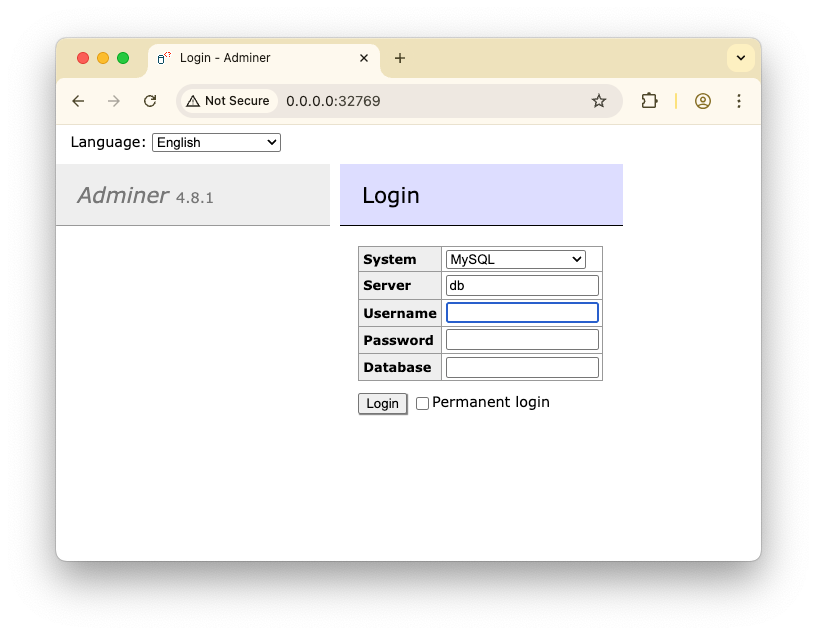
When to Use This Pattern
Use dynamic ports when:
- You're running multiple services that would otherwise conflict.
- You're using a reverse proxy (like Traefik or NGINX) to route traffic internally.
- The service is only being accessed by other containers (use Docker networking instead of exposing to host).
- You're in a CI environment where port conflicts are common and automation is key.
Pro Tip: Communicate Ports Internally
Rather than relying on host-exposed ports at all, leverage Docker’s internal DNS. One container can talk to another using the service name:
services:
web:
build: .
ports:
- 3000
api:
build: ./api
environment:
- WEB_URL=http://web:3000
This keeps traffic inside the Docker network and removes the need for exposed ports unless absolutely necessary.
Conclusion
Port conflicts are a common pain point when working with Docker Compose, especially in team environments or shared machines. Removing hardcoded host ports and letting Docker assign dynamic ones simplifies local development and makes your setup more robust.
Remember: unless a human needs to access the service directly, don’t expose the port.